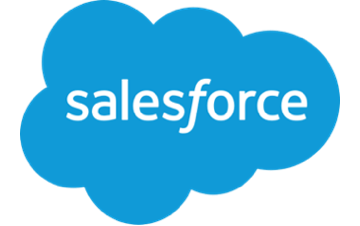
Hi All. Recently i came across a situation where we need to create a component in Lightning which should be visible in Salesforce 1 App as Menu Option. In that Component user can add Account Details and on click of Save it should save and redirect the user to created Record in SF1 itself. Today i thought of sharing the code and how to implement this in Salesforce.
Basic Introduction: For this functionality i have created a Lightning component that allow user to create a new Account and redirect to the record view screen. I have also created Lightning page that will be visible in SF1 app and in that page I have added my Lightning component.
Code Implementation :
1. I have create one lightning component that is responsible for creating the form, saving and redirecting user to View Record Page. In Lightning component i have one component file (.cmp) and one javascript controller file (.js). The code for component file is :
<aura:component implements="flexipage:availableForAllPageTypes" access="global" controller="CreateAccountCompController"> <aura:attribute name="newAccount" type="Account" default="{ 'sobjectType': 'Account','Name': ''}"/> <div class="container"> <form class="slds-form--stacked"> <div class="slds-form-element__control"> <ui:inputText aura:id="accName" label="Account Name" class="slds-input" labelClass="slds-form-element__label" value="{!v.newAccount.Name}" required="true" /> </div> <div class="slds-form-element__control"> <ui:inputPhone label="Phone" class="slds-input" labelClass="slds-form-element__label" value="{!v.newAccount.Phone}" /> </div> <div class="slds-form-element__control"> <ui:inputPhone label="Fax" class="slds-input" labelClass="slds-form-element__label" value="{!v.newAccount.Fax}" /> </div> <div class="slds-form-element__control"> <ui:inputEmail label="Email" class="slds-input" labelClass="slds-form-element__label" value="{!v.newAccount.Email}" /> </div> <div class="slds-form-element__control"> <ui:inputText label="Website" class="slds-input" labelClass="slds-form-element__label" value="{!v.newAccount.Website}" /> </div> <div class="slds-form-element"> <ui:button label="Submit" class="slds-button slds-button--neutral" press="{!c.createAccount}"/> </div> </form> </div> </aura:component>
Code for Javascript Controller is :
({ createAccount : function(component, event) { var accountName = component.find("accName").get("v.value"); if(!accountName || 0 === accountName.length || !accountName.trim()){ alert("Please Enter Account Name"); return; } var saveAction = component.get("c.saveAccount"); saveAction.setParams({ "account": component.get("v.newAccount") }); saveAction.setCallback(this, function(response) { if (component.isValid() && response.getState() === "SUCCESS") { var accountId = response.getReturnValue(); if(navigator.userAgent.match('(Mobi)')){ var sObectEvent = $A.get("e.force:navigateToSObject"); sObectEvent.setParams({ "recordId": accountId , "slideDevName": "detail" }); sObectEvent.fire(); }else{ window.location.href = '/'+ accountId; } } }); $A.enqueueAction(saveAction) } })
2. I have created on controller CreateAccountCompController that works with the component to insert Account record into database.
public class CreateAccountCompController { /* * @Param Account * @Return String * * This method takes Account as a Input and insert that account into database * Once inserted we are taking the AccountId from that account and returning back. */ @AuraEnabled public static String saveAccount(Account account){ insert account; return String.valueOf(account.Id); } }
3. Created App Page using Lightning App Builder.
Code Implementation :
To create this functionality I am using Lightning page that is visible in SF1 App. In that lightning page I am using Lightning component that allow users to create Account object. In Lightning component I am using Controller and Component for implementing this functionality. Along with that I am also using apex controller which is used to insert Account object in database. In Component of Lightning Component I am using attribute of type Account with default column value as blank, ui:inputText & ui:inputPhone & ui:inputEmail for taking input from user, ui:button to save the Account in database. All the input fields are binded with attribute Account object. On click on save button, I am calling JavaScript method written Controller of Lightning Component. In this Controller I am first of all checking if Account Name is blank or not, if it is blank I am showing alert message else continue. Then I am calling apex class aura enabled method and passing Account object as parameter to save the new object into database. From that apex class method I am returning the Account Id after insertion back to the JavaScript. In JavaScript we are using callback method which will get called when custom controller returns back. In this JavaScript I am able to access returned Account Id from apex class. Now with that Account Id I am redirecting user to view record page using navigationToSObject method for SF1 and window.location.href for desktop and laptop.
Screenshots :
Leave a Reply