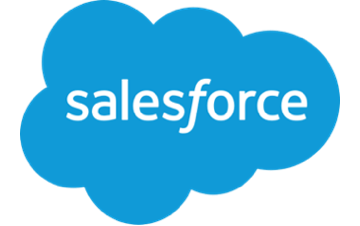
Hello guys. Recently i my company, i came across a situations where we need to create a visualforce page where user can add multiple contacts in one go. And on the same page, user can see all the contacts that is added by user with pagination option. Simple, correct ? Yes it is simple, but what makes this difficult is pagination. In pagination i have taken much time and finally implemented custom logic to make pagination work. I though of sharing the code and logic with your guys.
Basic Introduction: For this i have created 2 sections. In first section, I am showing contact fields in tabular format, save button and Add contact button. And in second section I am rendering all contacts which user has added from above section in tabular format with pagination functionality.
Code Implementation : For this i have created one visualforce page and 1 Apex Class. Code for Apex class is :
public class ContactsViewAddController{ public List<Contact> contactsToAdd{get;set;} public List<Contact> contactsToView{get;set;} public List<Contact> tempContacts{get;set;} public boolean isSaved {get;set;} /*This is configurable. We can show as much maximum records per page as we want*/ private final Integer maxRecordsPerPage = 3; public Integer totalNoOfRecords{get;set;} public List<String> pageNumberArray{get;set;} public String selectedPageNumber{get; set;} /* * Constructor * * To initialize List variables and adding blank new contact in "Add Contact" section. */ public ContactsViewAddController(){ contactsToAdd = new List<Contact>(); contactsToView = new List<Contact>(); tempContacts = new List<Contact>(); contactsToAdd.add(new Contact()); } /* * Save method get called when someone clicks on Save button in "Add Contact" Section. * * This method inserts the data in database, clears the data from "Add Contact" section table, *creates Page Number on the basis of data and shows the record in "View Contact" section */ public void save(){ insert contactsToAdd; ApexPages.addmessage(new ApexPages.message(ApexPages.Severity.INFO,'Data Saved Succesfully')); isSaved = true; tempContacts.clear(); tempContacts.addAll(contactsToAdd); contactsToAdd.clear(); contactsToAdd.add(new Contact()); totalNoOfRecords = tempContacts.size(); selectedPageNumber = '1'; createPageNumberArray(); changePage(); } /* * Add Contact method get called when someone clicks on Add Contact button in "Add Contact" Section. * * This method creates a new Contact object with no data and adds that to "Add Contact" Section table. */ public void addContact(){ contactsToAdd.add(new Contact()); } /* * createPageNumberArray is a supporting method that is called when someone saves the data in "Add Contact" Section * * This method creates a array of Page Number on the basis of Total Number of records we have to show and Max Number * of records per page. This method is used to create pagination commandLinks. */ public void createPageNumberArray(){ Double ceil =(Double) totalNoOfRecords / (Double)maxRecordsPerPage; Integer totalNoOfPages = (Integer)Math.ceil(ceil); System.debug(ceil+' '+totalNoOfPages+' '+totalNoOfRecords+' '+maxRecordsPerPage); pageNumberArray = new List<String>(); for(Integer i=1;i<=totalNoOfpages;i++){ pageNumberArray.add(i+''); } } /* * changePage method is used to create the content for "View Contact" Section on the basis of selected page number. */ public void changePage(){ contactsToView.clear(); Integer startIndex; Integer endIndex; Integer selectedPgNo = Integer.valueOf(selectedPageNumber); if(selectedPgNo == 1){ startIndex = 0; endIndex = maxRecordsPerPage; }else{ startIndex = (selectedPgNo-1)* maxRecordsPerPage; endIndex = (selectedPgNo) * maxRecordsPerPage; } if(endIndex>totalNoOfRecords){ endIndex = totalNoOfRecords; } for(Integer i= startIndex;i<endIndex;i++){ contactsToView.add(tempContacts.get(i)); } } }
and code for visualforce page is :
<apex:page controller="ContactsViewAddController" tabStyle="Contact"> <apex:form id="parentForm"> <apex:pageMessages /> <apex:pageBlock title="Add Contacts" id="addContactsDiv"> <apex:pageBlockTable value="{!contactsToAdd}" var="cont"> <apex:column headerValue="First Name"> <apex:inputField value="{!cont.FirstName}"/> </apex:column> <apex:column headerValue="Last Name"> <apex:inputField value="{!cont.LastName}"/> </apex:column> <apex:column headerValue="Phone"> <apex:inputField value="{!cont.Phone}"/> </apex:column> <apex:column headerValue="Email"> <apex:inputField value="{!cont.Email}"/> </apex:column> </apex:pageBlockTable> <apex:pageBlockButtons > <apex:commandButton action="{!save}" value="Save"/> <apex:commandButton action="{!addContact}" value="Add Contact" reRender="addContactsDiv"/> </apex:pageBlockButtons> </apex:pageBlock> <apex:pageBlock title="View Contacts" id="viewContactsDiv" rendered="{!isSaved}"> <apex:pageBlockTable value="{!contactsToView}" var="cont"> <apex:column headerValue="First Name" value="{!cont.FirstName}"/> <apex:column headerValue="Last Name" value="{!cont.LastName}"/> <apex:column headerValue="Phone" value="{!cont.Phone}"/> <apex:column headerValue="Email" value="{!cont.Email}"/> </apex:pageBlockTable> <apex:pageBlockButtons > <apex:repeat value="{!pageNumberArray}" var="pageNumber"> <a href="javascript:viewItem('{!pageNumber}');"> {!pageNumber} </a> </apex:repeat> <apex:actionFunction action="{!changePage}" name="viewItem" reRender="parentForm" immediate="true"> <apex:param name="selectedPgNo" value="" assignTo="{!selectedPageNumber}"/> </apex:actionFunction> </apex:pageBlockButtons> </apex:pageBlock> </apex:form> </apex:page>
Code Walkthrough: In this functionality I am using 2 pageBlockTable to showcase contact fields in a Tabular format, one is for adding Contacts and other one is for showing Contacts. When our apex class constructor is called, I am adding a new Contact object in the Add Contact List with no data so that when our UI gets rendered it will show a blank record in First Section pageBlockTable. When someone clicks on Add Contacts it adds another Contact object with no data in the List and refreshes the page. This way our UI shows 2 records. When someone clicks on save, I am inserting all thedata in the database in one go and renders the second pageBlock which will showcase all inserted Contacts. I have used custom logic to achieve pagination functionality. In UI Iam showing Page Numbers commandLinks, on click of that I am calling apex actionFunction which reads the value of clicked commandLink and set the value in our apex class variable and calls the apex method. In that method I am checking for the range that need to be visible in UI on the basis of selection and based on that I am filtering data from the List and refreshes the UI.
Screenshots :
When we open the page it will show 1 New Contact with 4 fields in Tabular format.
When some clicks on Add Contact, it will create new record for user to add more contacts.
When we click on Save it clears all data from Add Contact section and showcase those contacts in View Contacts grid with Pagination functionality.
Leave a Reply