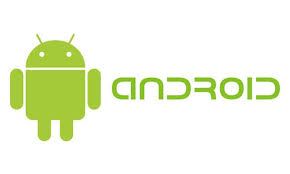
For passing values from one Activity to another activity you can use Intent’s Extras method.
What is Intent?
Intent is a way to switch from one activity to another. Syntax for Intent :
Intent intent = new Intent(MainActivity.this, new NewActivity()); startActivity(intent);
where first parameter of Intent constructor is the current Activity and second parameter is the New Activity which you want to open.
Now when you want to send data while switching from one Activity to another then you can use Extras method of Intent. That data can be in the form of String, Integer, Serialized object etc. So syntax to pass data along with your intent object is :
Intent intent = new Intent(MainActivity.this, new NewActivity()); intent.putExtras(“VALUE”,”THE_VALUE_IS_ENTERED_HERE”); startActivity(intent);
In the above example i am passing String data but you can pass Serialized object, Integer etc.
Now in another Activity (in our case NewActivity) you need to get values of Extras that we have passed with Intent. For that you can use syntax :
Bundle extras = getIntent().getExtras() String extrasValue = extras.getString("VALUE");
In the above code we are getting Bundles from Intent’s Extras. That intent can have multiple key value pairs as we can send as many as key value pairs in Extras method of Intent. Now i am using getString method and passing the key to retrieve the value of that key that should be a String value. And this method will return my String value (in my case it would be “THE_VALUE_IS_ENTERED_HERE” ;
Leave a Reply